In this post, I will show you step by step how you can easily develop a Keyword Research Tool similar to mine with the help of Google Suggestions Free API.
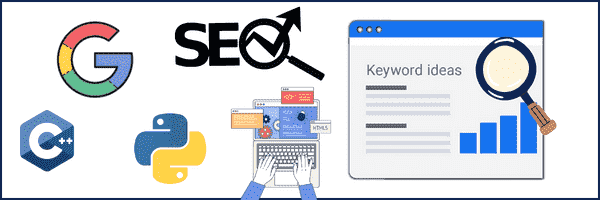
You can use keyword tools to:
- Find Content Ideas
- Explore Keywords To Rank On Search Engines.
- Or, best of all, sell it as a Subscription SaaS Product with some other features.
Last Week, I reverse-engineered 2 paid Big Keyword Research Tools to learn how they work and how you can build the same.
Even if you are not very experienced in programming, you can still easily follow up with this tutorial and develop your own keyword research tool with Python or C#.
So, what are those two websites?
How does “Answerthepublic.com” Work?
Answerthepublic.com is a website that allows users to type in a keyword or phrase and receive a list of related questions, prepositions, and long tail keywords that people have searched for on Google.
This can be useful for content creators who are looking for ideas for new articles, videos, or blog posts.
But, when you take a look at the pricing. You want to stay away from it! Don’t you?!
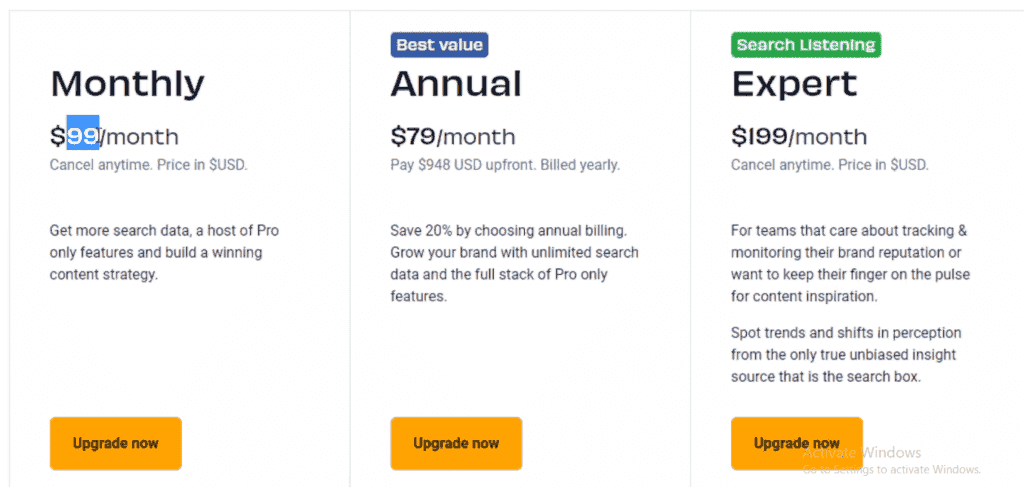
How does “Keywordtool.io” Work?
Keywordtool.io is a keyword research and analysis tool that helps users find and analyze the best keywords for their website or online business.
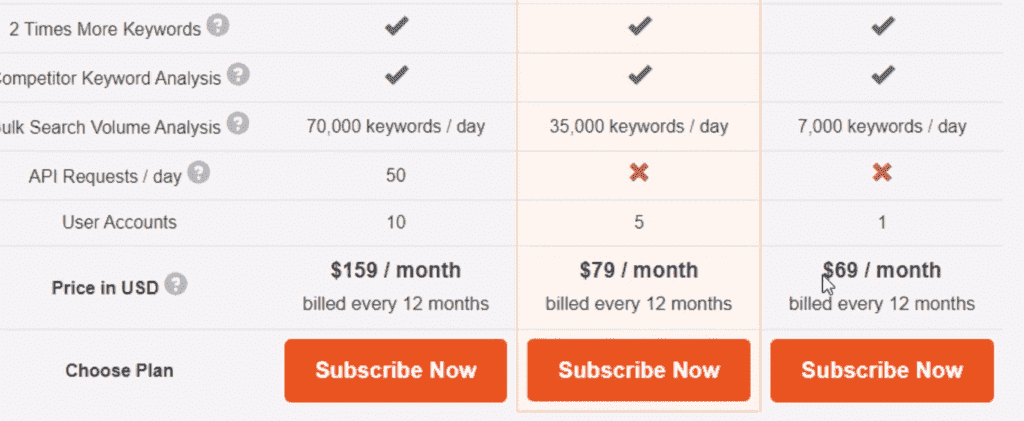
Both tools help you generate keyword ideas and suggestions. However, they are not available to everyone because of the heavy pricing we mentioned above.
So, if you want Your Own FREE Keyword research SEO tool, follow along, and let’s make it happen!
Here’s what we will cover:
- How Most Keyword Research / Keyword Idea Tools work
- How To Read Google Autosuggestions Programmatically?
- Develop a Keyword Research Tool in Python
- Develop a Keyword Research Tool in C#
How do Keyword Research Tools Work?
Well, it depends on the scope and functionality of the Tools you’re making, the complexity, and the SEO metrics you want your Tool to Provide.
In our case, we are providing “Google “Search” and “Question” Suggestions.
Let’s see how they are getting the results,
How do KeywordTool.io and AnswerthePublic.com get results for keyword suggestions?
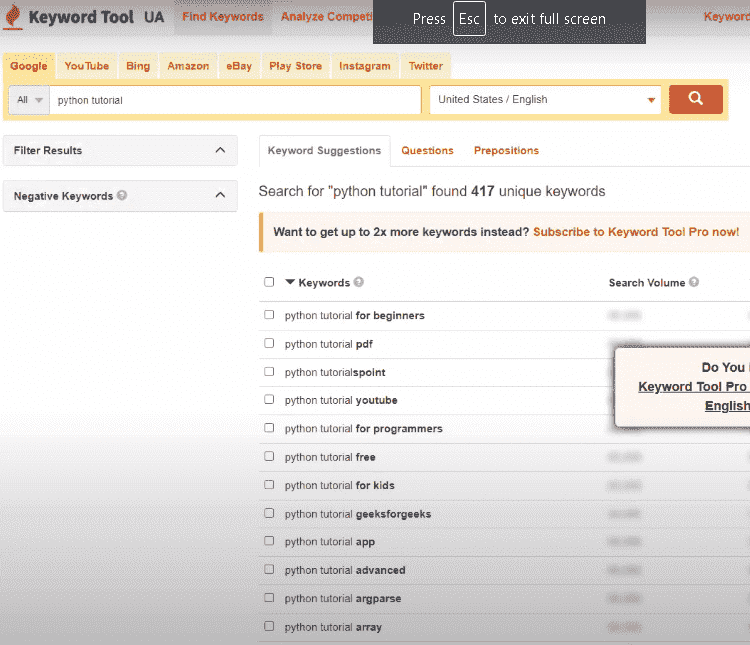
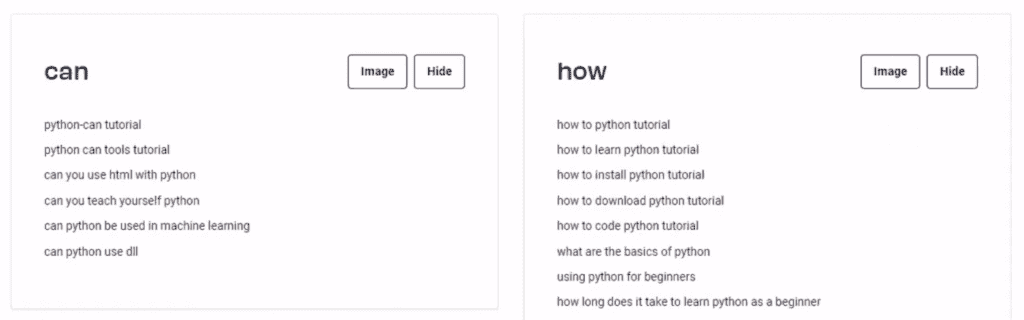
Let’s open Google and type the same query we type into KeywordTool.io
Type “Python Tutorial,” press space, and type the letter “f”. You will see Google suggests keywords, ideas, and search queries!
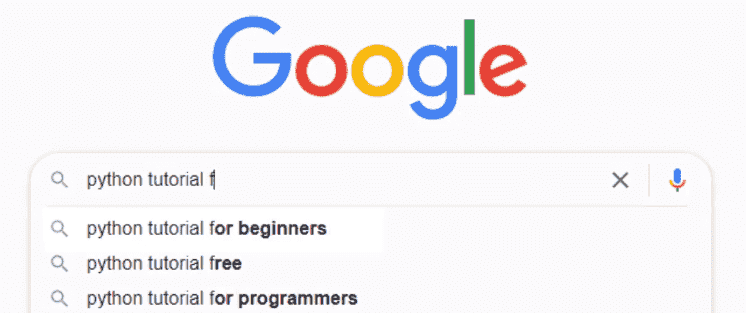
Now, try adding “how” before the “python tutorial” keyword in Google Search and see what Google suggests! As you can see we’re getting similar suggestions as the tools above.
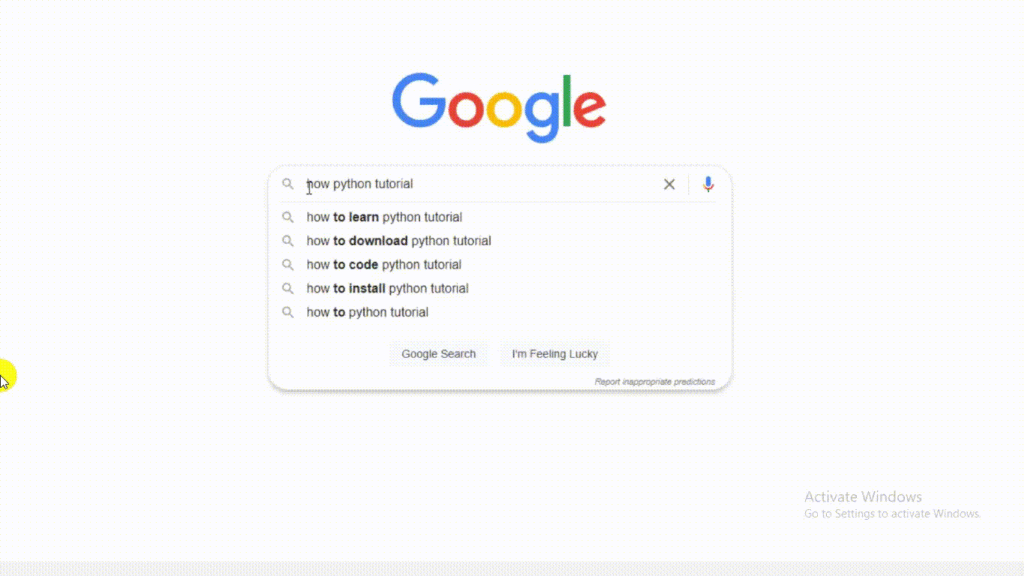
Now that we know how the whole process works, let’s see how to implement it from our code and Automate the Process to save you Time and Money.
How To Read Google Autosuggestions Programmatically?
Before we move on, I highly recommend you watch this short video to visualize the process and strengthen your concept. It is not obligatory; you can skip it and continue reading!
Mainly, We have two approaches:
- Using Web Automation and Scraping
- Using Google Search Free API Call
I chose the second approach simply because with web automation, the operation will be slower, and you may get Re-captcha to solve, and things will be more complicated.
API Call for Google Keyword Suggestions:
If you open your browser and paste this URL below, you will call the Google Suggestions API.
http://google.com/complete/search?output=toolbar&gl=COUNTRY&q=Your_QUERY
You can change the country and the query to get different Google suggestions in XML format using this call, as shown below:
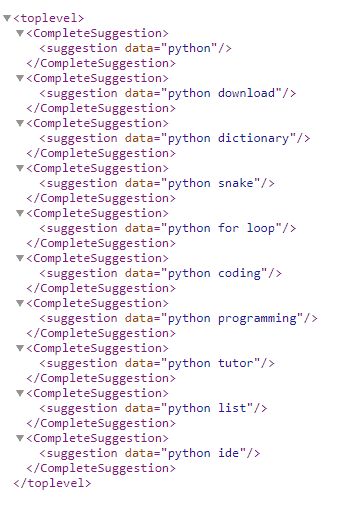
That’s it! We will call this URL from within the code and read the XML output!
To help more, I developed this tool in both C# .NET and Python. Both work the same way, so choose the one that you prefer!
Keyword Research Tool in Python
Here’s how the Python code will look like:
- First, we import requests (Used To Call API) and xml.etree.ElementTree (User To Read XML)
- Define a Class named QuestionsExplorer, and a method inside, GetQuestions, and it takes some arguments questionType, userInput, and countryCode to collect the Keywords and return them.
- Build the search URL based on user input. and call the API
- Read the API response results.
- Loop Through the XML results. And append them to the QuestionResults Array.
Keyword Research Tool in C#
I Tried To Implement The Code in the same way as in Python. First, we create the class, and it has a method.
<pre class="EnlighterJSRAW" data-enlighter-language="csharp" data-enlighter-theme="" data-enlighter-highlight="" data-enlighter-linenumbers="" data-enlighter-lineoffset="" data-enlighter-title="" data-enlighter-group="">//start class QuestionsExplorer { public List<string> GetQuestions(string questionType, string userInput, string countryCode) //end</pre>
Then, we create an object of the QuestionExplorer class in the Program’s Main Method, which is called the GetQuestion method.
<pre class="EnlighterJSRAW" data-enlighter-language="generic" data-enlighter-theme="" data-enlighter-highlight="" data-enlighter-linenumbers="" data-enlighter-lineoffset="" data-enlighter-title="" data-enlighter-group="">//start using System; using System.Collections.Generic; using System.Net.Http; using System.Xml.Linq; namespace GoogleSuggestion { //Define Class class QuestionsExplorer { public List<string> GetQuestions(string questionType, string userInput, string countryCode) { var questionResults = new List<string>(); //List To Return //Build Google Search Query string searchQuery = questionType + " " + userInput + " "; string googleSearchUrl = "http://google.com/complete/search?output=toolbar&gl=" + countryCode + "&q=" + searchQuery; //Call The URL and Read Data using (HttpClient client = new HttpClient()) { string result = client.GetStringAsync(googleSearchUrl).Result; //Parse The XML Documents XDocument doc = XDocument.Parse(result); foreach (XElement element in doc.Descendants("CompleteSuggestion")) { string question = element.Element("suggestion")?.Attribute("data")?.Value; questionResults.Add(question); } } return questionResults; } } class Program { static void Main(string[] args) { //Get a Keyword From The User Console.WriteLine("Enter a Keyword:"); var userInput = Console.ReadLine(); //Create Object of the QuestionsExplorer Class var qObj = new QuestionsExplorer(); //Call The Method and pass the parameters var questions = qObj.GetQuestions("what", userInput, "us"); //Loop over the list and pring the questions foreach (var result in questions) { Console.WriteLine(result); } //Finish Console.WriteLine(); Console.WriteLine("---Done---"); Console.ReadLine(); } } } //end</pre>
Get the full code in the below GitHub link:
Conclusion
In this post, I showed you how to build a keyword research tool based on Google’s Suggestion API using two popular programming languages: C# and Python.
You may wonder how you can improve it and build a tool like mine with search metrics like CPC, Monthly Search Volume, and Competition. Here’s a free guide that will help you!
Take care, and Happy Coding!
Wow. I did not know that building a keyword tool would be that easy. Kudos to you Hasan!
your welcome 🙂
It’s very helpful for me as a website designer and content writer. I am going to build my own keyword research tool soon with this tutorial and help others too via it! Thank you, Hasan for the resoruces and I am a subscriber of your Youtube channel and the Chatgpt prompt engineering course by you have made my life easier and my professional career is on Cloud-9 after I learnt it! Thanks!
Great! thanks
Hello,
Your videos Are awesome and would like to know that can we run source code with any hosting provider like godady ans hostinger for creating keyword tool for users
this requires a full tutorial, I will try my best to create one
Hello Hasan Sir ,
Your videos are much much worth it. Can you please create a step by step video for creating front end and backend tool using any API keys for creating too having help of webflow , bubble and netlify ( no code tools ) which will help for targeting users use to get to know more information…about their questions..
I just love your content, especially learning Python please add more if you have time 🙂
Python is very important nowadays, and becomes a must wanted in terms of knowledge and basics….
Sir, how can I use this source code in WordPress, please tell me sir. This is very important for me at this time.
you need to convert it into API
Sir, please tell me how this happens. Is there a tutorial about this on Google? I have written the name of this project in college with your trust. 🥺🙏
I made it
Hi Hasan. I have created a keyword research tool. now according to your code , i am trying to create a keyword suggestion tool but dont you think , this is illegal and google will ban my website for directly accessing like this instead of using Google Custom Search Engine implemention? Can you guide? Thanks
it is used by most of the tools, I have been using it for years
Can i create keyword research tool in javascrpt
yes you can