How To Build & Sell Tiny AI Agents: A Step-by-Step Guide
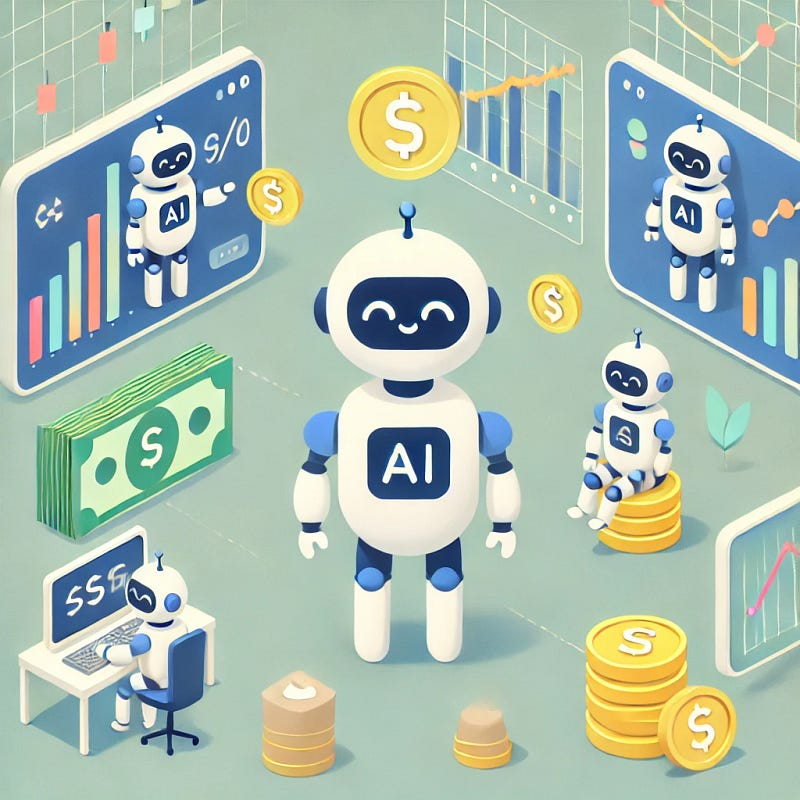
Today, there’s a great opportunity to build and monetize small, focused AI agents that deliver high value without the complexity of full-scale AI autonomous systems.
In this post, I’ll walk you through exactly how to build these “Tiny AI agents” and turn them into profitable online digital products.
You will turn each Single AI Agent into 3 Income streams!
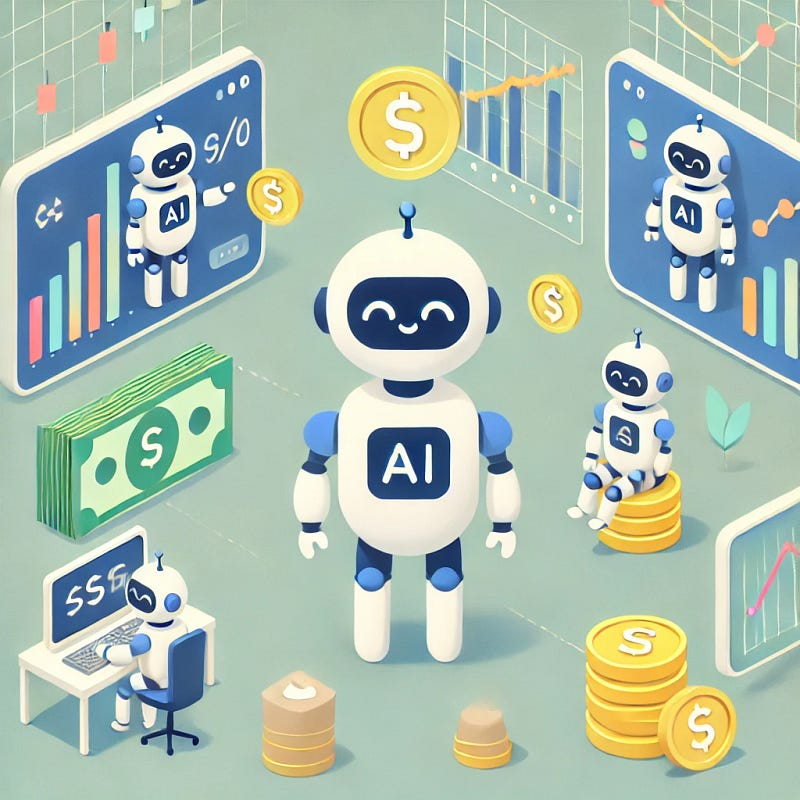
What Are Tiny AI Agents?
Before diving into the technical details, let’s understand what I mean by “tiny AI agents.”
A tiny AI agent is a specialized AI system pre-built to perform specific actions based on defined parameters and inputs.
Unlike comprehensive AI systems, these agents are:
- Focused on a single task — They do one thing extremely well
- Quick to develop — You can build one in a few hours
- High-value — They solve real problems for users
- Easy to monetize — They can be packaged into various digital products
While some might argue these are just “AI workflows” rather than true agents (since they lack fully autonomous reasoning), the terminology matters less than what they can do: deliver value quickly and effectively.
If you’re interested in building true autonomous agents from scratch (with full reasoning capabilities), check out my in-depth guide.
The Core Engine: LLMÂ Router
The secret ingredient powering these tiny agents is what I call an “LLM Router” — a decision-making component that selects the best action path based on inputs.
I recently added this functionality to my open-source Python package, SimplerLLM, which makes building these agents straightforward even if you’re not an AI expert.
The LLM Router works by:
- Directing the workflow accordingly
- Taking user input
- Analyzing it against a set of possible choices or templates
- Selecting the optimal path for processing that input
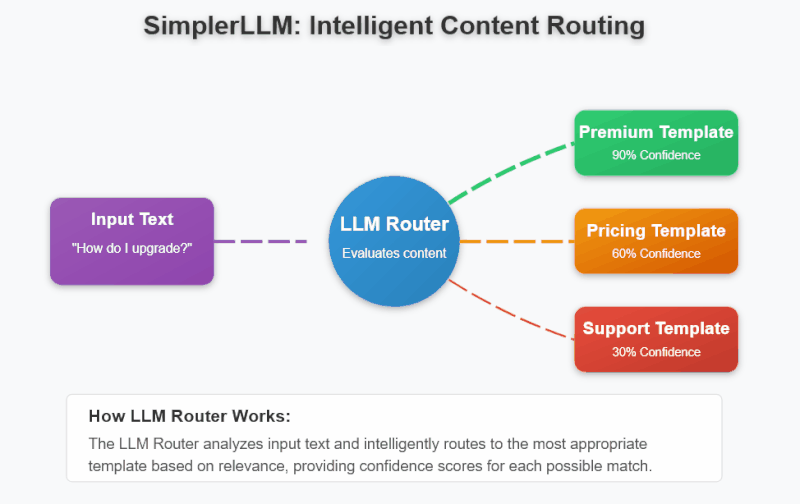
This approach allows a tiny agent to make intelligent decisions without requiring complex autonomous reasoning systems.
Building A Real-World Tiny AI Agent: Tweet Generator
Let’s build a practical example: a tweet generator that takes content from various sources (YouTube videos, blog posts, or direct text) and creates engaging tweets in your personal style.
This agent has three core components:
- Content Input — What the tweets will be about
- Templates — Tweet structures proven to perform well
- Profile — Your personal style, background, and voice
Step 1: Set Up The Core Components
First, let’s import the necessary libraries and set up our environment:
# Import necessary libraries import re import requests from typing import List from enum import Enum from pydantic import BaseModel from bs4 import BeautifulSoup # Import SimplerLLM libraries from SimplerLLM.language.llm_addons import generate_pydantic_json_model_async from SimplerLLM.language.llm_router import LLMRouter from templates import get_template_choices
Step 2: Define Our Models
Next, we’ll define the structure for our input sources and output:
# Define source types class SourceType(str, Enum): """ Enum for different types of content sources. - YOUTUBE: Content from a YouTube video (will extract transcript) - BLOG: Content from a blog post (will scrape paragraphs) - TEXT: Direct text input """ YOUTUBE = "youtube" BLOG = "blog" TEXT = "text" # Define the tweet output model using Pydantic class TweetOutput(BaseModel): """ Pydantic model for generated tweets output. - tweets: List of generated tweets """ tweets: List[str]
Step 3: Extract Content From Different Sources
Our agent needs to be flexible in accepting content from various sources:
async def extract_content(source_type: SourceType, content: str) -> str: """ Extract content from different sources. Parameters: - source_type: Type of content source (YouTube, blog, or text) - content: The content identifier (URL or text) Returns: - Extracted content as a string """ # Case 1: If source is YouTube, extract the transcript if source_type == SourceType.YOUTUBE: # Extract the video ID from the YouTube URL match = re.search(r"(?:youtube\.com/watch\?v=|youtu\.be/|youtube\.com/shorts/)([\w-]+)", content) if not match: raise ValueError("Invalid YouTube URL format") video_id = match.group(1) # Get the transcript using the video ID transcript = await get_youtube_transcript(video_id) return transcript # Case 2: If source is a blog, scrape the paragraphs elif source_type == SourceType.BLOG: # Make a request to the blog URL response = requests.get(content) # Parse the HTML content soup = BeautifulSoup(response.text, 'html.parser') # Extract all paragraphs and join them return " ".join([p.text for p in soup.find_all('p')]) # Case 3: If source is direct text, return it as is elif source_type == SourceType.TEXT: return content # Default case: If source type is not recognized, return the content as is return content
Step 4: Implement The LLM Router For Template Selection
This is where the magic happens – the router analyzes the content and selects the most appropriate tweet templates:
def select_best_templates(content: str, llm_instance) -> List[dict]: """ Select the best templates for the given content using LLMRouter. Parameters: - content: The content to generate tweets from - llm_instance: The language model instance Returns: - List of selected templates with their metadata """ # Create a new LLMRouter instance router = LLMRouter(llm_instance=llm_instance) # Add template choices to the router router.add_choices(get_template_choices()) # Create a prompt that instructs the LLM to select appropriate templates selection_prompt = f""" Please analyze the following content and select the most appropriate tweet templates that would work well for creating engaging tweets about this topic: CONTENT: {content} Select templates that: 1. Match the tone and subject matter of the content 2. Would help highlight the key points effectively 3. Would resonate with the target audience for this topic """ # Get the best 3 templates using the router top_templates = router.route_top_k_with_metadata( selection_prompt, # Using the instructional prompt k=3, # Select top 3 templates metadata_filter={} # No filtering ) # Extract the selected templates with their metadata selected_templates = [] for result in top_templates: template_index = result.selected_index template_content, template_metadata = router.get_choice(template_index) selected_templates.append({ "template": template_content, "metadata": template_metadata }) return selected_templates
Step 5: Generate Tweets Based On Content, Templates, And Profile
Finally, we use the selected templates and profile to generate personalized tweets:
async def generate_tweets(content: str, templates: List[dict], llm_profile: str, llm_instance) -> TweetOutput: """ Generate tweets based on content, templates, and LLM profile using SimplerLLM. Parameters: - content: The content to generate tweets from - templates: List of selected templates with their metadata - llm_profile: The LLM profile text - llm_instance: The language model instance Returns: - TweetOutput object with generated tweets """ # Create a prompt for the LLM prompt = f""" # TASK: Generate 3 Viral X Tweets You are a social media expert specializing in creating high-engagement Twitter content. ## CONTENT TO Create Tweets based on: ``` {content} ``` ## TEMPLATES TO FOLLOW Use these templates as structural guides for your Tweets. Each Tweet should follow one of these templates: ``` {templates[0]['metadata']['format']} {templates[1]['metadata']['format']} {templates[2]['metadata']['format']} ``` ## LLM PROFILE (Your background and style) ``` {llm_profile} ``` ## REQUIREMENTS - Create exactly 3 Tweets, each following one of the provided templates - Each Tweet must be around 8-10 tweets under 280 characters each - Include relevant hashtags where appropriate (max 2-3) - Focus on providing value or insights that would make users want to share - Adapt the style to match the LLM profile ## OUTPUT FORMAT Provide 3 ready-to-post Tweets that follow the templates and capture the essence of the content. """ # Generate tweets using SimplerLLM's pydantic model generation response = await generate_pydantic_json_model_async( model_class=TweetOutput, prompt=prompt, llm_instance=llm_instance ) return response
Step 6: Putting It All Together
Our main function orchestrates the entire workflow:
async def main(source_type: SourceType, content: str, llm_profile_path: str, llm_instance): """ Main function to demonstrate the tweet generation flow. Parameters: - source_type: Type of content source (YouTube, blog, or text) - content: The content identifier (URL or text) - llm_profile_path: Path to the LLM profile text file - llm_instance: The language model instance """ # 1. Extract content from the source print(f"Step 1: Extracting content from {source_type.value} source...") extracted_content = await extract_content(source_type, content) # 2. Select the best templates using LLMRouter print("\nStep 2: Selecting best templates with LLMRouter...") templates = select_best_templates(extracted_content, llm_instance) # 3. Read the LLM profile print("\nStep 3: Reading LLM profile...") llm_profile = read_llm_profile(llm_profile_path) # 4. Generate tweets using SimplerLLM print("\nStep 4: Generating tweets with SimplerLLM...") tweet_output = await generate_tweets(extracted_content, templates, llm_profile, llm_instance) # 5. Display the results print("\nGenerated Tweets:") for i, tweet in enumerate(tweet_output.tweets, 1): print(f"\nTweet {i}:") print(tweet)
With just these few components, we’ve built a functional AI agent that:
- Takes content from multiple sources
- Analyze it to select appropriate templates
- Generates personalized tweets in your voice
- Returns ready-to-use content
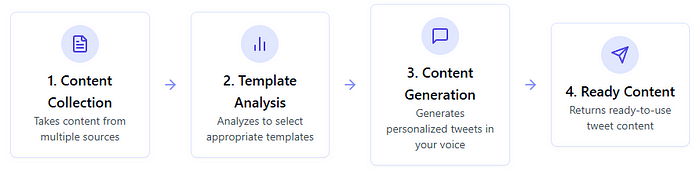
Get the Complete Code on GitHub
Want to explore the full implementation and start building your own tiny agents right away?
Check out the complete code repository on GitHub:
The repository includes:
- The full code
- Additional template examples
- Documentation
Star the repository if you find it useful, and don’t forget to share what you build with it!
3 Ways to Monetize Your Tiny AI Agents
Now that you’ve built a valuable tiny AI agent, let’s explore three different methods to turn it into income streams:
1. Turning Your Agent into an API
One of the most straightforward ways to monetize your agent is by transforming it into an API that others can integrate into their workflows. Platforms like RapidAPI make this process simple:
- Package your agent as an API endpoint
- List it on RapidAPI’s marketplace
- Set up tiered pricing (free tier for discovery, paid tiers for volume)
- Collect recurring revenue as developers use your API
I’ve published several APIs this way and continue to earn passive income from them. The beauty of APIs is that they allow other developers to integrate your agent’s capabilities into their own products.
Check out this video, for example, to see in action how to build and Sell APIs even without coding:
2. Building a SaaS on WordPress
Another powerful monetization approach is turning your agent into a full Software-as-a-Service (SaaS).
This might sound complex, but with WordPress, it’s remarkably straightforward:
- Set up a WordPress website with a membership plugin
- Create an interface for your AI agent
- Implement a token/credit system for usage
- Market your tool to potential users
I’ve done this with PromoterKit.com, which offers a set of AI-powered marketing tools, including image generators, SEO tools, and content creation assistants.
I’ve also built TubeDigest, which is still in beta but shows how easily these agents can be packaged as user-friendly tools on top of WordPress!
The SaaS model gives you:
- Recurring subscription revenue
- Direct relationship with your customers
- Opportunity to expand with additional tools
3. Creating a Chrome Extension
The third monetization path is building a Chrome extension that brings your agent’s functionality directly into users’ browsers:
- Package your agent’s functionality as a browser extension
- List it on the Chrome Web Store
- Monetize through premium features or subscriptions
Extensions are particularly powerful because they integrate directly into users’ existing workflows, making your agent’s capabilities available exactly when needed.
I’ve built several extensions, including one that allows users to generate AI-powered replies to social media comments with a single click. The development process took just a few hours, but the value delivered to users is substantial.
Check out the guide below to see how I Created A Chrome Extension With AI in 60 Minutes! (And You Can Do The Same)
The Key to Success: Take Action Now
If you’re thinking, “This sounds great, but I’m a beginner and can’t build something like this” — I need you to hear this clearly:
We are in 2025, at the beginning of a new AI era. If you keep saying “I’m a beginner,” you’ll remain a beginner forever.
Today, you have unlimited access to tools, learning resources, and AI assistance that makes building these agents accessible to anyone willing to put in the effort.
My challenge to you:
Dedicate just ONE HOUR a day to learning and experimenting with AI. In 30 days
You’ll be amazed at what you can build.
Instead of scrolling endlessly through social media or binging another Netflix series, invest that time in building skills that can transform your future.
With tools like SimplerLLM, the barrier to entry has never been lower.
Get Started Today!
The complete code for the tweet generator agent is available for free, along with detailed documentation. You can download it, run it, modify it, and build your own agents based on this template.
If you need help along the way, join our public forum, where I’m active almost daily, answering questions and helping community members overcome challenges.
The opportunity is here now, but it won’t last forever. Those who take action early will have a significant advantage in this new AI economy.
Next Steps
- Download the sample agent code and run it on your computer
- Experiment with different templates and profiles
- Choose one of the monetization paths and start building
- Dedicate at least one hour daily to developing your skills
Want to stay updated with my latest AI development insights and opportunities?Â
Subscribe to my newsletter for weekly guides, code examples, and unique digital business strategies.
What tiny AI agent will you build? Let me know in the comments below!
Responses