Ai Affiliate marketing Chatbot on WordPress – Frontend
🔑 ID:
67283
👨💻
HTML
🕒
29/10/2024
Free
Description:
This code is used to build the AI Chatbot for promoting affiliate products, as shown in this guide:
https://learnwithhasan.com/promote-affiliate-products-with-ai-chatbots/
Code:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="style.css"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.2.0/css/all.min.css" /> <script src="https://unpkg.com/@dotlottie/player-component@latest/dist/dotlottie-player.mjs" type="module"></script> <script defer> const apiUrl = 'https://YourWebsite.com/wp-json/myapi/v1/test-aff-chat-bot/'; //change the URL to match your site const botConfigurationUrl = 'https://learnwithhasan.com/wp-json/myapi/v1/test-aff-chat-bot-config'; const copyButtons = document.querySelectorAll('.lwh-open-cbot .copy-button'); const button = document.querySelector('.lwh-open-cbot #submit-btn'); let messageInput = document.querySelector('.lwh-open-cbot #message'); let content = []; let botConfigData = ''; let conversationTranscript = []; function lwhOpenCbotToggleChat() { const chatWindow = document.querySelector(".lwh-open-cbot .chat"); chatWindow.classList.toggle('show'); if(chatWindow.classList.contains('show')){ document.querySelector(".lwh-open-cbot .custom-chatbot").style.zIndex = '9999' } else{ document.querySelector(".lwh-open-cbot .custom-chatbot").style.zIndex = '9998' } } function lwhOpenCbotonFormSubmit(event, userMessage) { event.preventDefault(); if(button.disabled == true) return let message; if(userMessage == undefined){ message = messageInput.value.trim(); document.querySelector(".lwh-open-cbot .startup-btns").style.display = "none"; } else{ message = userMessage; } content.push({ role: 'user', message: message, }); let timestamp = new Date().toLocaleString(); conversationTranscript.push({ sender: 'user', time: timestamp, message: message, }); data = ""; if (message !== '') { lwhOpenCbotaddMessage('user', message); messageInput.value = ''; lwhOpenCbotaddTypingAnimation('ai'); lwhOpenCbotfetchData() } } function lwhOpenCbotaddMessage(sender, message) { let chatMessagesContainer = document.querySelector('.lwh-open-cbot .chat__messages'); let messageContainer = document.createElement('div'); messageContainer.classList.add('chat__messages__' + sender); let messageDiv = document.createElement('div'); messageDiv.innerHTML = ` ${sender === 'ai' ? ` <div> <img width="30px" class="bot-image" src="${botConfigData.botImageURL}" alt="bot-image"> </div> ` : "" } <p>${message} </p> ${sender === 'user' ? ` <div> <img width="30px" class="avatar-image" src="${botConfigData.userAvatarURL}" alt="avatar"> </div> ` : "" } `; messageContainer.appendChild(messageDiv); chatMessagesContainer.appendChild(messageContainer); chatMessagesContainer.scrollTop = chatMessagesContainer.scrollHeight; } function lwhOpenCbotaddTypingAnimation(sender) { let chatMessagesContainer = document.querySelector('.lwh-open-cbot .chat__messages'); let typingContainer = document.createElement('div'); typingContainer.classList.add('chat__messages__' + sender); let typingAnimationDiv = document.createElement('div'); typingAnimationDiv.classList.add('typing-animation'); typingAnimationDiv.innerHTML = ` <div> <img width="30px" class="bot-image" src="${botConfigData.botImageURL}" alt=""> </div> <p> <svg height="16" width="40" style="max-height: 20px;"> <circle class="dot" cx="10" cy="8" r="3" style="fill:grey;" /> <circle class="dot" cx="20" cy="8" r="3" style="fill:grey;" /> <circle class="dot" cx="30" cy="8" r="3" style="fill:grey;" /> </svg> </p> `; typingContainer.appendChild(typingAnimationDiv); chatMessagesContainer.appendChild(typingContainer); chatMessagesContainer.scrollTop = chatMessagesContainer.scrollHeight; } function lwhOpenCbotreplaceTypingAnimationWithMessage(sender, message) { let chatMessagesContainer = document.querySelector('.lwh-open-cbot .chat__messages'); let typingContainer = document.querySelector('.lwh-open-cbot .chat__messages__' + sender + ':last-child'); if (typingContainer) { let messageContainer = document.createElement('div'); messageContainer.classList.add('chat__messages__' + sender); messageContainer.classList.add('chat_messages_ai'); let messageDiv = document.createElement('div'); messageDiv.innerHTML = ` ${sender === 'ai' ? ` <div> <img width="30px" class="bot-image" src="${botConfigData.botImageURL}" alt="bot-image"> </div> ` : "" } <p class="typing-effect"></p> ${sender === 'user' ? ` <div> <img width="30px" class="avatar-image" src="${botConfigData.userAvatarURL}" alt="avatar"> </div> ` : "" } `; messageContainer.appendChild(messageDiv); typingContainer.parentNode.replaceChild(messageContainer, typingContainer); const typingEffectElement = messageDiv.querySelector(".typing-effect"); let index = 0; const typingInterval = setInterval(() => { typingEffectElement.textContent += message[index]; index++; if (index === message.length) { clearInterval(typingInterval); typingEffectElement.insertAdjacentHTML('beforeend', `<span title="copy" class="copy-text" onclick="lwhOpenCbotcopyText(event)"><i class="fa-regular fa-copy"></i><span>copied</span></span>`); chatMessagesContainer.scrollTop = chatMessagesContainer.scrollHeight; } }, 5); chatMessagesContainer.scrollTop = chatMessagesContainer.scrollHeight; } } function lwhOpenCbotremoveTypingAnimation() { let typingAnimationDivs = document.querySelectorAll('.lwh-open-cbot .typing-animation'); typingAnimationDivs.forEach(function (typingAnimationDiv) { let chatMessagesAiDiv = typingAnimationDiv.closest('.chat__messages__ai'); if (chatMessagesAiDiv) { chatMessagesAiDiv.parentNode.removeChild(chatMessagesAiDiv); } }); } copyButtons.forEach(button => { button.addEventListener('click', function (event) { const codeElement = this.parentNode.nextElementSibling.querySelector('code'); const codeText = codeElement.textContent; navigator.clipboard.writeText(codeText).then(function () { event.target.innerText = "Copied"; setTimeout(() => { event.target.innerText = "Copy"; }, 2000); }).catch(function (error) { console.error('Error copying code: ', error); }); }); }); function lwhOpenCbotcopyText(event) { const paragraph = event.target.closest('p'); const clone = paragraph.cloneNode(true); clone.querySelectorAll('.copy-text').forEach(elem => { elem.parentNode.removeChild(elem); }); const textToCopy = clone.textContent.trim(); navigator.clipboard.writeText(textToCopy) .then(() => { const copiedSpan = event.target.nextElementSibling; copiedSpan.style.display = 'block'; setTimeout(() => { copiedSpan.style.display = 'none'; }, 2000); }) .catch(error => { console.error('Error copying text: ', error); }); } async function lwhOpenCbotfetchData() { button.disabled = true; try { response = await fetch(apiUrl, { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ "last_prompt": content[content.length - 1].message, "conversation_history": content.map(item => ({ "role": item.role, "content": item.message })) }) }); if (response.ok) { data = await response.json(); console.log(data, "Data from api"); button.disabled = false; if (!data.success) { lwhOpenCbotremoveTypingAnimation() lwhOpenCbotshowPopup('Oops! Something went wrong!', '#991a1a'); throw new Error("Something went wrong. Please Try Again!"); } } else { lwhOpenCbotshowPopup('Oops! Something went wrong!', '#991a1a'); throw new Error("Request failed. Please try again!"); } content.push({ role: 'assistant', message: data.result, }); let timestamp = new Date().toLocaleString(); conversationTranscript.push({ sender: 'bot', time: timestamp, message: data.result, }); lwhOpenCbotreplaceTypingAnimationWithMessage('ai', data.result); } catch (error) { lwhOpenCbotremoveTypingAnimation(); lwhOpenCbotshowPopup('Oops! Something went wrong!', '#991a1a'); console.error('There was a problem with the fetch operation:', error); return; } } async function lwhOpenCbotfetchBotConfiguration() { const chatMessagesContainer = document.querySelector(".lwh-open-cbot .chat__messages"); document.querySelector(".lwh-open-cbot .loading").style.display = 'flex'; chatMessagesContainer.innerHTML = ''; let startupBtnsDiv = document.createElement('div'); startupBtnsDiv.classList.add('startup-btns'); chatMessagesContainer.appendChild(startupBtnsDiv); let botResponse = '' try { botResponse = await fetch(botConfigurationUrl); if (botResponse.ok) { botConfigData = await botResponse.json(); console.log(botConfigData, "Data from api"); chatMessagesContainer.style.fontSize = `${botConfigData.fontSize}px`; let startupBtns='' const startupBtnContainer = document.querySelector('.lwh-open-cbot .startup-btns'); botConfigData.commonButtons.forEach(btn => { startupBtns += `<p onclick="lwhOpenCbotonFormSubmit(event, '${btn.buttonPrompt}'); lwhOpenCbothandleStartupBtnClick(event);">${btn.buttonText}</p>`; }); startupBtnContainer.innerHTML = startupBtns; if (botConfigData.botStatus == 1) { document.querySelector(".lwh-open-cbot .chat__status").innerHTML = `<span></span> Online`; document.querySelector(".lwh-open-cbot .chat__status").querySelector("span").style.background = "#68D391"; } document.querySelector(".lwh-open-cbot .loading").style.display = 'none'; lwhOpenCbotaddMessage('ai', botConfigData.StartUpMessage); content.push({ role: 'assistant', message: botConfigData.StartUpMessage, }); let timestamp = new Date().toLocaleString(); conversationTranscript.push({ sender: 'bot', time: timestamp, message: botConfigData.StartUpMessage, }); } else { document.querySelector(".lwh-open-cbot .loading").style.display = 'none'; lwhOpenCbotshowPopup('Oops! Something went wrong!', '#991a1a'); throw new Error("Request failed. Please try again!"); } } catch (error) { lwhOpenCbotshowPopup('Oops! Something went wrong!', '#991a1a'); button.disabled = true console.error('There was a problem with the fetch operation:', error); return; } } function lwhOpenCbotshowPopup(val, color) { button.disabled = false; const popup = document.querySelector('.lwh-open-cbot .popup'); popup.style.display = 'block'; popup.style.opacity = 1; const innerPopup = popup.querySelector('p'); innerPopup.innerText = val; innerPopup.style.color = color; popup.classList.add('popup-animation'); setTimeout(() => { popup.classList.remove('popup-animation'); popup.style.display = 'none'; popup.style.opacity = 0; }, 3000); } function lwhOpenCbothandleStartupBtnClick(event){ const startupBtnContainer = event.target.parentNode; startupBtnContainer.style.display = 'none'; } lwhOpenCbotfetchBotConfiguration(); </script> <title>Mini Chatbot</title> </head> <body> <div class="chatbot-container lwh-open-cbot"> <div class="custom-chatbot__image" onclick="lwhOpenCbotToggleChat()"> <dotlottie-player src="https://lottie.host/feefeb87-8206-4b50-8696-bda4747b4fdf/uUJIo0ICb6.json" background="transparent" speed="1" style="width: 80px; height: 80px;" loop autoplay></dotlottie-player> </div> <div class="custom-chatbot"> <div class="chat"> <div class="feedback-form"> <div class="feedback-header"> <p>Feedback</p> <p class="feedback__modal-close" onclick="lwhOpenCbotremoveFeedbackModal()"><i class="fa-solid fa-xmark"></i></p> </div> <form onsubmit="lwhOpenCbotsendFeedback(event)"> <textarea name="feedback" id="feedback" rows="4" required></textarea> <button type="submit">Send Feedback</button> </form> </div> <div class="loading"> <p><i class="fa-solid fa-circle-notch fa-spin"></i></p> <p>Wait a moment</p> </div> <div class="popup"> <p>Oops! Something went wrong!</h2> </div> <div class="chat__header"> <div> <div class="chat__title"> AI Chatbot </div> <div> <div class="chat__status"><span></span> Offline</div> </div> </div> <div> <div class="chat__close-icon" onclick="lwhOpenCbotToggleChat()"> <i class="fa-solid fa-xmark"></i> </div> </div> </div> <div class="chat__messages"> </div> <div class="chat__input-area"> <div class="selected-image-container"> </div> <form id="messageForm" onsubmit="lwhOpenCbotonFormSubmit(event)"> <div> <div class="input"> <div> <input type="text" id="message" name="message" placeholder="Type your message" autocomplete="off" required> </div> </div> <div> <button type="submit" id="submit-btn"><i class="fa-solid fa-paper-plane"></i></button> </div> </div> </form> </div> </div> </div> </div> </body> </html>
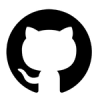
GitHub Link
✖️ Not Available
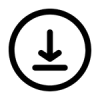
Download File
✖️ Not Available
If you’re encountering any problems or need further assistance with this code, we’re here to help! Join our community on the forum or Discord for support, tips, and discussion.