Ai Affiliate marketing Chatbot on WordPress – Backend
🔑 ID:
67280
👨💻
PHP
🕒
29/10/2024
Free
Description:
This code is used to build the AI Chatbot for promoting affiliate products, as shown in this guide:
https://learnwithhasan.com/promote-affiliate-products-with-ai-chatbots/
Code:
function test_aff_generate_chat_response( $last_prompt, $conversation_history ) { $json_string = '[ { "unqiue_service_name": "Hostinger", "starting_price": "$2.99/month", "description": "Hostinger offers Managed WordPress Hosting with multiple plans to suit different needs, making it easier to launch a professional online presence.", "features": "WordPress multisite support, 100 domain-based email addresses, WordPress themes, Over 100 subdomains", "uptime_guarantee": null, "security_features": null, "backup_options": null, "free_domain": "Yes, a free domain name registration is included in WordPress plans.", "money_back_guarantee": "Yes, a 30-day money-back guarantee is provided.", "details": "Free domain registration includes .com extension. You can create up to 100 domain-based email addresses at no extra cost.", "affiliate_link": "https://affiliate.link/hostinger" }, { "unqiue_service_name": "SiteGround", "starting_price": null, "description": "SiteGround offers three ways to transfer a WordPress website: manual migration by experts, self-transfer, or using the free WordPress Migrator plugin.", "features": "Manual migration by SiteGround experts, self-transfer for tech-savvy users, and a free WordPress Migrator plugin for easy migration.", "uptime_guarantee": null, "security_features": null, "backup_options": null, "free_domain": null, "money_back_guarantee": null, "details": "The WordPress Migrator plugin is free and easy to use, allowing users to transfer their WordPress websites quickly.", "affiliate_link": "https://affiliate.link/siteground" }, { "unqiue_service_name": "GreenGeeks", "starting_price": null, "description": "GreenGeeks offers a secure, high-performance platform optimized for WordPress websites with eco-friendly hosting, offsetting 300% of the energy consumption with renewable energy credits.", "features": "1-click WordPress installation, automatic WordPress updates, advanced tools like WP Staging, WP-CLI, and Git, high-performance technologies including SSD hard drives, LiteSpeed Web Server, MariaDB database servers, PHP 8, HTTP/2, LSCache technology, CloudFlare CDN, 24/7 WordPress expert support, free WordPress site migration services.", "uptime_guarantee": null, "security_features": "Security features are included in the shared hosting plans, but specific details are not mentioned.", "backup_options": null, "free_domain": null, "money_back_guarantee": null, "details": "GreenGeeks is an eco-friendly web host, offsetting 300% of the energy your site uses through renewable energy credits. The service includes a staging option for testing before moving a WordPress site. WordPress installations are automatically updated with previous versions available if needed.", "affiliate_link": "https://affiliate.link/greengeeks" } ]'; $affiliate_system_prompt = "You are an expert in wordpress hosting services, I will provide you with a [JSON_DATA_FILE] containing a list of services with their features and details. Your task is to keep questioning the user based on the [JSON_DATA_FILE] till you know the best service to suggest for him. IMPORTANT: Make sure to ask multiple questions for better user experience. Ask one question at a time. Make sure Return the affiliate link with your suggestion. [JSON_DATA_FILE]: $json_string"; // OpenAI API URL and key $api_url = 'https://api.openai.com/v1/chat/completions'; $api_key = 'sk-proj-UOHkGB0IJK0uZsExEn5IT3BlbkFJyKgDofOZAgR9zvNSBvhR'; // Replace with your actual API key // Headers for the OpenAI API $headers = [ 'Content-Type' => 'application/json', 'Authorization' => 'Bearer ' . $api_key ]; // Add the last prompt to the conversation history $conversation_history[] = [ 'role' => 'system', 'content' => $affiliate_system_prompt ]; $conversation_history[] = [ 'role' => 'user', 'content' => $last_prompt ]; // Body for the OpenAI API $body = [ 'model' => 'gpt-4o', // You can change the model if needed 'messages' => $conversation_history, 'temperature' => 0.7 // You can adjust this value based on desired creativity ]; // Args for the WordPress HTTP API $args = [ 'method' => 'POST', 'headers' => $headers, 'body' => json_encode($body), 'timeout' => 120 ]; // Send the request $response = wp_remote_request($api_url, $args); // Handle the response if (is_wp_error($response)) { return $response->get_error_message(); } else { $response_body = wp_remote_retrieve_body($response); $data = json_decode($response_body, true); if (json_last_error() !== JSON_ERROR_NONE) { return [ 'success' => false, 'message' => 'Invalid JSON in API response', 'result' => '' ]; } elseif (!isset($data['choices'])) { return [ 'success' => false, 'message' => 'API request failed. Response: ' . $response_body, 'result' => '' ]; } else { $content = $data['choices'][0]['message']['content']; return [ 'success' => true, 'message' => 'Response Generated', 'result' => $content ]; } } } function test_aff_handle_chat_bot_request( WP_REST_Request $request ) { $last_prompt = $request->get_param('last_prompt'); $conversation_history = $request->get_param('conversation_history'); $response = test_aff_generate_chat_response($last_prompt, $conversation_history); return $response; } function test_aff_load_chat_bot_base_configuration(WP_REST_Request $request) { // You can retrieve user data or other dynamic information here $user_avatar_url = "https://learnwithhasan.com/wp-content/uploads/2023/09/pngtree-businessman-user-avatar-wearing-suit-with-red-tie-png-image_5809521.png"; // Implement this function $bot_image_url = "https://learnwithhasan.com/wp-content/uploads/2023/12/site-logo-mobile.png"; // Implement this function $response = array( 'botStatus' => 1, 'StartUpMessage' => "Hi, How are you?", 'fontSize' => '16', 'userAvatarURL' => $user_avatar_url, 'botImageURL' => $bot_image_url, // Adding the new field 'commonButtons' => array( array( 'buttonText' => 'Pick Hosting Service', 'buttonPrompt' => 'I need help picking a hosting service please.' ) ) ); $response = new WP_REST_Response($response, 200); return $response; } add_action( 'rest_api_init', function () { register_rest_route( 'myapi/v1', '/test-aff-chat-bot/', array( 'methods' => 'POST', 'callback' => 'test_aff_handle_chat_bot_request', 'permission_callback' => '__return_true' ) ); register_rest_route('myapi/v1', '/test-aff-chat-bot-config', array( 'methods' => 'GET', 'callback' => 'test_aff_load_chat_bot_base_configuration', )); } );
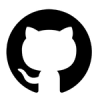
GitHub Link
✖️ Not Available
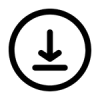
Download File
✖️ Not Available
If you’re encountering any problems or need further assistance with this code, we’re here to help! Join our community on the forum or Discord for support, tips, and discussion.