AI Router
🔑 ID:
63674
👨💻
Python
🕒
18/08/2024
Free
Description:
This code is part of a tutorial I posted on my blog.
It takes an input query from the user, and it gives them the most suitable API to use in their case from a customized list of APIs.
Code:
import json import time from SimplerLLM.language.llm import LLM, LLMProvider from SimplerLLM.tools.generic_loader import load_content def fetch_apis(filepath): text_file = load_content(filepath) content = json.loads(text_file.content) return content def find_best_api(user_query, apis): llm_instance = LLM.create(provider=LLMProvider.OPENAI, model_name="gpt-4o-mini") u_prompt = f""" You are an expert in problem solving. I have a user specific query and I want to check if I have an API that would help him solve this problem. I'll give you both the user inquery and the list of APIs in the inputs section delimited between triple backticks. So analyze both of them very well and check if there's an API which can help him or no. ##Inputs user inquiry: ```[{user_query}]``` API list: ```[{apis}]``` #Output The output should only be the API name as provided in the inputs and nothing else. If no API was found return None. """ response = llm_instance.generate_response(prompt=u_prompt) return response # Input: filepath = 'apis.json' apis = fetch_apis(filepath) user_query = input("Enter your inquiry: ") start_time = time.time() # Start timer result = find_best_api(user_query, apis) if result!="None": print(f"\nThe best API to use is: {result}\n") else: print("\nNo suitable API found.\n") end_time = time.time() # End timer print(f"Execution time: {end_time - start_time} seconds")
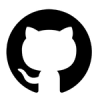
GitHub Link
✖️ Not Available
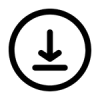
Download File
✖️ Not Available
If you’re encountering any problems or need further assistance with this code, we’re here to help! Join our community on the forum or Discord for support, tips, and discussion.